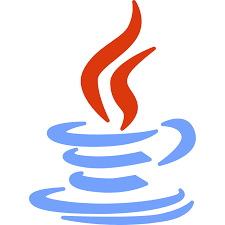
Learning Java
Start Date: 28-Mar-2020
End Date: 27-April-2020(expected)
Operators
Operators in Java are special symbols that perform specific operations on one, two or three operands and then return a result.
What is an Operand?
An operand is a term used to describe any object that is manipulated by an operator. If we consider below statement:
int myVar = 15 + 12;
Then + is the operator, and 15 and 12 are operands. Variables used instead of literals are also operands.
double mySalary = hoursWorked * hourlyRate;
In the above line hoursWorked and hourlyRate are operands, and * (multiplication) is the operator.
What is an Expression?
An expression is formed by combining variables, literals, method and return values and operators.
In the line below, 15 + 12 is the expression which has (or returns) 27 in this case.
int myVar = 15 + 12;
Summary:
double mySalary = hoursWorked * hourlyRate;
int myVar = 15 + 12; |
|
+ * | are Operator |
15, 12, hoursWorked, hourlyRate | are Operand |
15 + 12 and hoursWorked * hourlyRate | are Expressions |
What is a Comment?
Comments '//' are ignored by the computer and are added to a program to help describe something. Comments are there for humans. Aside from describing something about a program, comments can be used to temporarily disable code.
if-then Statements in Java
The if-then statement is the most basic of all control flow statements. It tells the program to execute a certain section of code only if a particular test evaluates to true. This is known as conditional logic.
Conditional Logic
Conditional logic uses specific statements in Java to allow us to check a condition and execute certain code based on whether that condition (the expression) is true or false.
Info! if-then rule always uses a code block. A code block allows more than one statement to be executed - a block of code.
Code | Output |
---|---|
Without Code Block
|
And I'm scared of Alien. |
Now with Code Block
|
It is not an Alien. And I'm scared of Alien. |
Java Operator Precedence Table
Larger number means higher precedence. Link
Precedence | Operator | Type | Associativity |
---|---|---|---|
15 | () [] · |
Parentheses Array subscript Member selection |
Left to Right |
14 | ++ -- |
Unary post-increment Unary post-decrement |
Right to left |
13 | ++ -- + - ! ~ ( type ) |
Unary pre-increment Unary pre-decrement Unary plus Unary minus Unary logical negation Unary bitwise complement Unary type cast |
Right to left |
12 | * / % |
Multiplication Division Modulus |
Left to right |
11 | + - |
Addition Subtraction |
Left to right |
10 | << >> >>> |
Bitwise left shift Bitwise right shift with sign extension Bitwise right shift with zero extension |
Left to right |
9 | < <= > >= instanceof |
Relational less than Relational less than or equal Relational greater than Relational greater than or equal Type comparison (objects only) |
Left to right |
8 | == != |
Relational is equal to Relational is not equal to |
Left to right |
7 | & | Bitwise AND | Left to right |
6 | ^ | Bitwise exclusive OR | Left to right |
5 | | | Bitwise inclusive OR | Left to right |
4 | && | Logical AND | Left to right |
3 | || | Logical OR | Left to right |
2 | ? : | Ternary conditional | Right to left |
1 | = += -= *= /= %= |
Assignment Addition assignment Subtraction assignment Multiplication assignment Division assignment Modulus assignment |
Right to left |
Summary of Operators:
Simple Assignment Operator
= Simple assignment operator
Arithmetic Operators
+ Additive operator (also used for String concatenation) - Subtraction operator * Multiplication operator / Division operator % Remainder operator
Unary Operators
+ Unary plus operator; indicates positive value (numbers are positive without this, however) - Unary minus operator; negates an expression ++ Increment operator; increments a value by 1 -- Decrement operator; decrements a value by 1 ! Logical complement operator; inverts the value of a boolean
Equality and Relational Operators
== Equal to != Not equal to > Greater than >= Greater than or equal to < Less than <= Less than or equal to
Conditional Operators
&& Conditional-AND
|| Conditional-OR
?: Ternary (shorthand for
if-then-else statement)
Type Comparison Operator
instanceof Compares an object to a specified type
Bitwise and Bit Shift Operators
~ Unary bitwise complement << Signed left shift >> Signed right shift >>> Unsigned right shift & Bitwise AND ^ Bitwise exclusive OR | Bitwise inclusive OR
Official Link
Operator Examples:
Abbreviating Operators: |
|
= result++; |
---|---|---|
Logical && Operator |
|
The AND operator comes in two flavors in Java, as does the Or
operator. && is the Logical AND which operates on boolean
operands - Checking if a given condition is true or false. The & is a bitwise operator working at the bit level. |
Logical || Operator |
|
Likewise || is the Logical OR which again operates
on boolean operands - checking if a given condition is true
or false. Again, | is a bitwise operator working at the bit level. |
The ! Operator | The ! or NOT Operator is also known as the Logical Compliment Operator. For use with booleans it tests the alternative value - as we can see below (isCar) tests for true, by adding a ! operator before the value we can check the opposite - false in this case. |
boolean isCar = false; We can use either of these statements: if (isCar == false) if (!isCar) to check if the boolean is Car is false. |
? : Ternary Operator |
boolean wasCar = isCar ? true : false; In this case we have three operands viz: isCar, true, false. isCar: The first operand is the condition we are testing. It evaluates if it is true or false. True: if the value assigned to 'wasCar' i.e., first condition was true. False: if the value assigned to 'wasCar' i.e, first condition was false. If isCar = false -> no output will be there.
int ageOfClient = 20;
In this case, isEighteenOrOver is assigned the value true because ageOfClient has the value 20. It can be a good idea to use parenthesis like this to make the code more readable. boolean isEighteenOrOver = (ageOfClient == 20) ? true : false; |
The ternary operator is a shortcut to assigning one of two values to a variable
depending on a given condition. It's a shortcut of the if-then-else statement. int ageOfClient = 20; boolean isEighteenOrOver = ageOfClient == 20 ? true : false; Operand one - ageOfClient == 20 in this case is the condition we're checking. It needs to return true or false. Operand two - true here is the value of assign to the variable isEighteenOrOver if the condition above is true Operand three - false here is the value to assign to the variable isEighteenOrOver if the condition above was false |
Coding Examples:
Testing boolean condition (= vs ==)
* (copy and paste in editor to see the error)
|
|
Other Ways or representing this condition
|
Operator Precedence & Operator Challenge
* 1. Create a double variable with a value of 20.00.
* 2. Create a second variable of type double with the value 80.00.
* 3. Add both numbers together and multiply by 100.00.
* 4. Use the remainder operator to figure out what the remainder from the result of
* the operation in step 3 and 40.00.
* We used the modulus or remainder operator on ints, but we can also use it on double.
* 5. Create a boolean variable that assigns the value true if the remainder( %) in #4 is 0,
* of false if it is not zero.
* 6. Output the boolean variable.
* 7. Write an 'if-then' statement that displays a message "Got some remainder" if the boolean
* in #5 is not true.
package challanges;
public class OperatorsChallange {
public static void main(String[] args) {
double firstValue = 20.00d;
double secondVariable = 80.00d;
double operation = (firstValue + secondVariable) * 100.00d;
//if not using parenthesis, then operator precedence will follow
System.out.println(operation);
double remainder = operation % 40.00d;
System.out.println(remainder);
boolean output = (remainder == 0) ? true : false; ///// HIGHLIGHTED pay attention
System.out.println(output);
if (!output) {
System.out.println("Got Some Remainder!!!");
}
/*
* if(remainder == 0) { output = true; }
* else { output = false; }
* boolean finalOutput = output? true : false;
* if (!finalOutput) { System.out.println("Got Some Remainder!!!"); }
*/
}
}